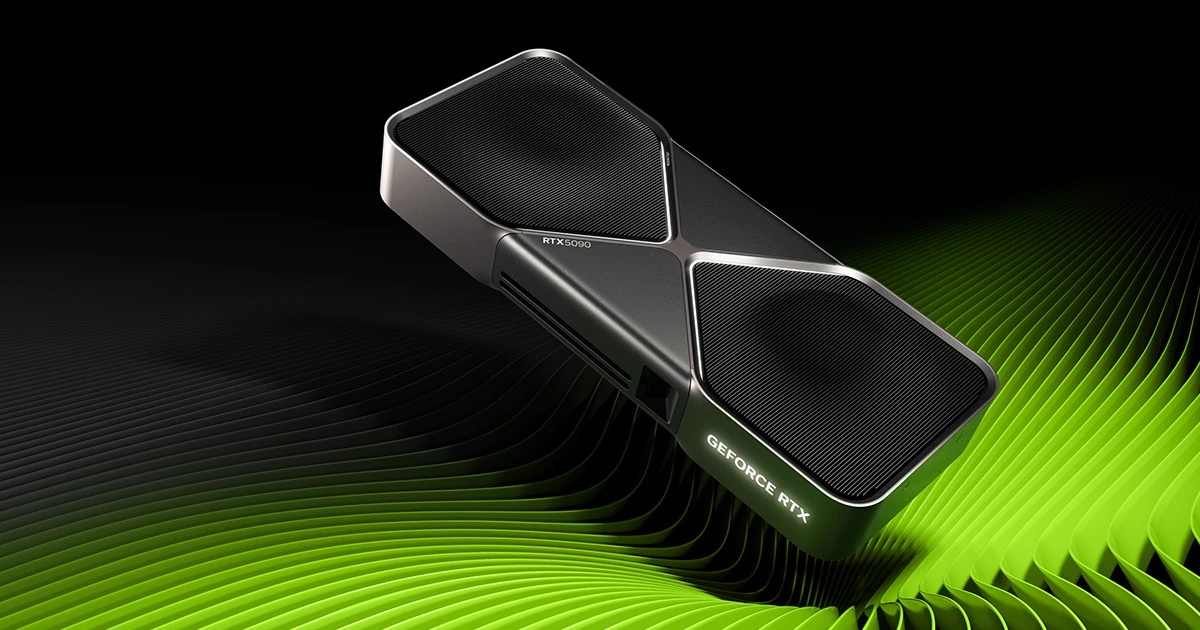
Introduction
Although client-side rendering of web interfaces is currently very popular, Django with “batteries” provides extensive functionality for server-side rendering, which allows you to quickly implement interfaces that solve business problems.
In this article, I want to talk about existing approaches to rendering web forms in Django.
Problem
For demonstration we will use a simple model:
# models.py
class Customer(models.Model):
name = models.CharField(max_length=50)
address = models.CharField(max_length=200)
cust_number = models.IntegerField(blank=False, null=False)
city = models.CharField(max_length=50)
state = models.CharField(max_length=50)
zipcode = models.CharField(max_length=10)
To work with forms, Django provides the forms module, which includes meta classes that allow you to declaratively generate a form for our model:
# forms.py
class CustomerForm(forms.ModelForm):
class Meta:
model = Customer
fields = '__all__'
Let's create a simple view to demonstrate the resulting forms:
# views.py
class CustomerCreateView(CreateView):
model = Customer
form_class = CustomerForm
Let's add the form to the html page template:
# customer_form.html
{% extends 'base.html' %}
{% block content %}
{{ form }}
{% endblock %}
Congratulations! We needed exactly 4 lines of code to render the form including many fields and one line to add it to the template (not counting the creation of the http endpoint). But you must admit, in the current version the form looks extremely ascetic:
❌ No stylization of forms
✅ The form is generated automatically
Django doesn't yet know that we're using the Bootstrap 5 CSS framework (although you can use any other) to style the HTML pages.
Naive solution
Bootstrap provides a wide range of classes and ready-made HTML snippets for use in form templates. The first solution that comes to mind is: “Let's add the necessary elements directly to the page template!”
In this case, we take care of writing the html code of the form, using snippets provided by the css library. In this way, you can achieve flawless form stylization while controlling each element individually. But there are also disadvantages to this approach. From one line like:
{{ form }}
, the form grows to tens and even hundreds of lines. We are forced to control widgets, labels, tooltips, validation error output, and much more. At the same time, reuse and support with this approach are extremely difficult, since the form is created individually, for a specific implementation.
✅ We control the styling and template of the form
❌ We write an html form template manually for a specific implementation
Something is wrong here, because at the previous stage we used the built-in Django form rendering mechanisms, let's not abandon them!
Labor-intensive solution
To comply with the DRY principle and keep the logic of the forms in the appropriate classes, let's consider a different approach.
According to the documentation for applying styles to a Bootstrap element
<input>
must have a class
form-label
. We can override the fields generated by the forms.ModelForm meta class using class variables, initializing widgets for them with the necessary attribute, for example:
# class CustomerForm
name = forms.CharField(
widget=forms.TextInput(attrs={'class': 'form-control'}),
)
True, then, we need to define all the attributes of the field ourselves, because the generated field object will override the automatically generated one. Therefore, it is better to extend the method
__init__
as shown below:
# forms.py
class CustomerForm(forms.ModelForm):
class Meta:
model = Customer
fields = '__all__'
def __init__(self, *args, **kwargs):
super().__init__(*args, **kwargs)
for field in self.fields.values():
field.widget.attrs['class'] = 'form-control'
We use the built-in mechanisms for generating declared fields by calling the parent method
__init__()
, after which, in a loop for each field widget, add a key-value
'class': 'form-control'
to the dictionary
widget.attrs
. Django will use the attribute
attrs
when rendering an element
<input>
, this way we will get the desired result.
If you style an element
<input>
is not enough, and it is necessary to change the html structure of the form, then you can resort to another tool. Meta classes of forms in Django allow you to override the templates used for rendering, using class variables of the form template_name_* (for example template_name_div or template_name_label depending on the template being overridden). This way we can abandon defining the html code of a specific form implementation in favor of overriding reusable templates.
The templates supplied with the framework are stored in the following directory:
venv/Lib/site-packages/django/forms/templates/django/forms
.
Bootstrap uses div-based form layout, so we’ll take the new template as a basis
forms/div.html
. Let's change the template to match the Bootstrap snippet:
# div.html
...
{% for field, errors in fields %}
<div {% with classes=field.css_classes %}class="form-floating mb-3 {{ classes }}"{% endwith %}>
{{ errors }}
{{ field }}
{% if field.use_fieldset %}
<fieldset>
{% if field.label %}{{ field.legend_tag }}{% endif %}
{% else %}
{% if field.label %}{{ field.label_tag }}{% endif %}
{% endif %}
{% if field.help_text %}<div class="form-text">{{ field.help_text|safe }}</div>{% endif %}
{% if field.use_fieldset %}</fieldset>{% endif %}
{% if forloop.last %}
{% for field in hidden_fields %}{{ field }}{% endfor %}
{% endif %}
</div>
{% endfor %}
...
Thus, an excellent result was obtained; we kept the page template minimalistic and encapsulated the form rendering in the appropriate class, while using the built-in form generation mechanisms for Django models.
✅ We control the styling and template of the form
✅ Generate HTML template automatically
❌ Missing form submit button and html attributes
action
And
method
(defined in the template)
But there are also disadvantages: we are forced to create new templates for specific implementations of forms. As is often the case, the Python community offers a ready-made solution for advanced form rendering in Django.
Ready solution
One of the most popular solutions for rendering forms in Django is the package django-crispy-forms (4.9k stars on GitHub). The package allows you to connect and use ready-made template libraries for various front-end frameworks, for example Bootstrap , tailwind , Bulma and etc.
Crispy provides powerful tools for generating all kinds of shape representations. You can find out more about all the features of crispy in official documentation . Here I will just give an example of a form from a real project:
# forms.py
class DecisionMakerForm(forms.ModelForm):
class Meta:
model = DecisionMaker
fields = '__all__'
class CustomerForm(forms.ModelForm):
FIELD_GROUPS = {
'main': ('status', 'inn', 'name'),
'decision_maker': (*DecisionMakerForm().fields.keys(), 'source'),
'shipment': ('total_volume', 'target_volume', 'current_supplier',
'consumed_items', 'problematic', 'purchase_method'),
'other': ('note', ),
}
class Meta:
model = Customer
fields = '__all__'
def __init__(self, *args, form_action: Optional[str] = None, **kwargs):
super().__init__(*args, **kwargs)
status = forms.ModelChoiceField(
initial=CustomerStatus.objects.first(),
required=True,
queryset=CustomerStatus.objects.all(),
label=self._meta.model.status.field.verbose_name,
)
self.fields['status'] = status
# Добавляем поля DecisionMakerForm
self.fields.update(DecisionMakerForm().fields)
# Добавляем атрибут helper для рендеринга формы с помощью crispy
self.helper = FormHelper()
self.helper.form_id = 'id-customer-form'
self.helper.form_method = 'post'
self.helper.form_action = form_action
self.helper.layout = Layout(
Fieldset('', *self.FIELD_GROUPS['main']),
Accordion(AccordionGroup('ЛПР', *self.FIELD_GROUPS['decision_maker']),
AccordionGroup('Поставки', *self.FIELD_GROUPS['shipment']),
css_class='mb-3'),
Fieldset('', *self.FIELD_GROUPS['other']),
Submit('submit', 'Сохранить', css_class='float-end'),
)
In this example, when initializing the form object, we add the attribute
helper
which refers to a class object
FormHelper
provided by the package
crispy
. Using a class
FormHelper
we can define html attributes of an element
<form>
, such as the method and url used when submitting the form. Thus, we encapsulate all the logic of the form into the appropriate class without scattering its parts among html templates.
But the class included in the library is of greatest interest
Layout
. This is a truly powerful tool for form template configuration. By combining python objects that display bootstrap snippets, we can declare complex form templates, leaving the work of rendering the html code to the algorithms.
In the example we use field groups (
Fieldset()
), bootstrap accordion with two tabs (objects
Accordion()
And
AccordionGroup()
) even a confirmation button (
Submit()
). In this case, in the template we only define the location of the form, and we define all the logic associated with it in the corresponding class:
# customer_form.html
{% extends 'base.html' %}
{% load crispy_forms_tags %}
{% block content %}
<div class="col-4">
{% crispy form %}
</div>
{% endblock %}
✅ We control the styling and template of the form
✅ Generate HTML template automatically
✅ Declaring the form submit button and attributes
action
And
method
Conclusion
The choice of approach depends on the requirements of the project and the preferences of the developer. However, using ready-made solutions such as django-crispy-forms can greatly simplify the process of developing and maintaining forms, while providing DRY, flexibility, control over the styling and structure of the form.