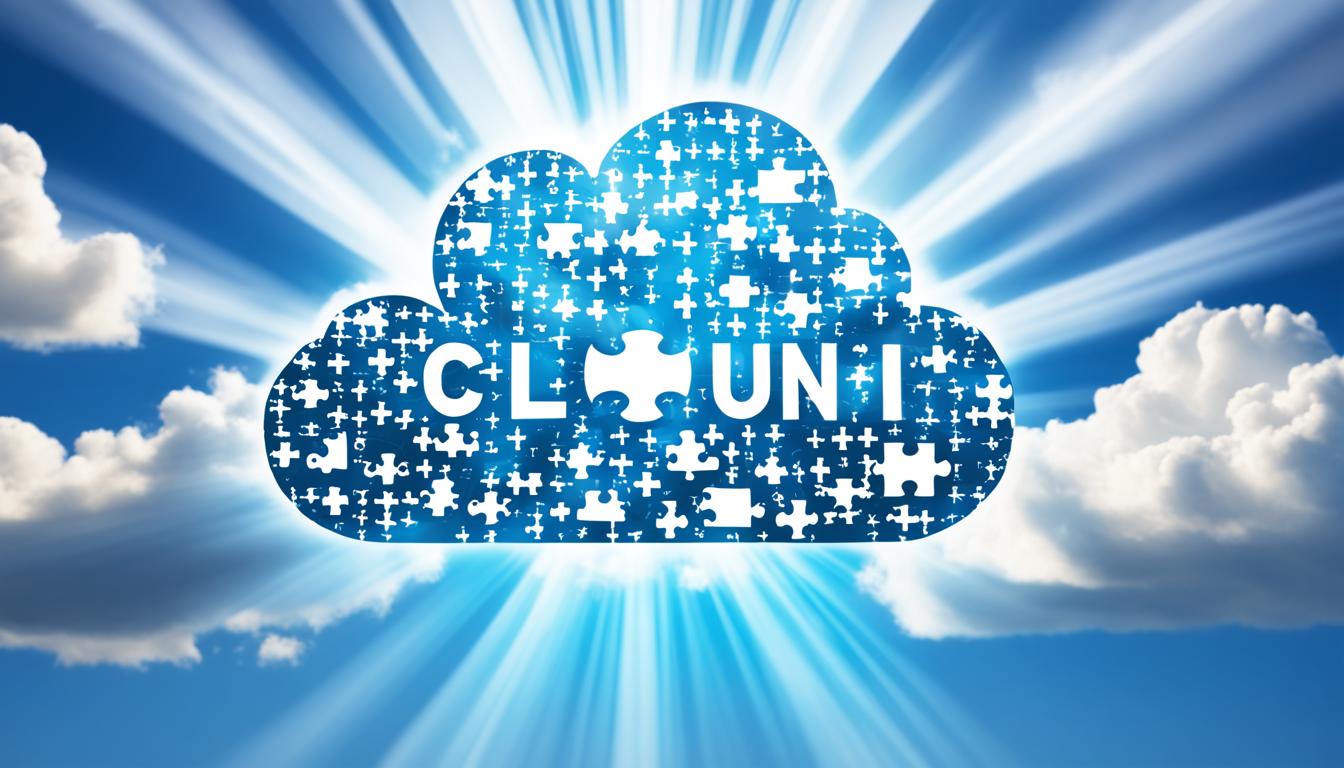
Figuring out what Python error message s mean can be quite challenging when you're first learning the language. Here is a list of common errors that can cause your program to crash.
Omission ":" after the operator if, elif, else, for, while, class or def. (Error message: " SyntaxError : invalid syntax ")
Example code with error:
if
spam
== 42
print
('Hello!')
Using = instead of ==. (Error message: "SyntaxError: invalid syntax" )
= is an assignment operator, and == is an equals comparison operator.
Example code with error :
if spam = 42:
print('Hello!')
Using the wrong amount of indentation. (Error message: "IndentationError: unexpected indent " And "IndentationError: unindent does not match any outer indentation level" And "IndentationError: expected an indented block" )
Remember that indentation increases only after a statement ending with a ":" colon, and must subsequently revert to the previous indentation.
Example code with error:
print('Hello!')
print('Howdy!')
… more:
if spam == 42:
print('Hello!')
print('Howdy!')
… more:
if spam == 42:
print('Hello!')
Forget to call len() in a loop statement for . (Error message: "TypeError: 'list' object cannot be interpreted as an integer" )
If you want to iterate over the indices of elements in a list or string , this requires calling the function range () . Just remember to pass the return value len(someList) instead of transferring only someList.
Example code with error:
spam = ['cat', 'dog', 'mouse']
for i in range(spam):
print(spam[i])
Correct option :
spam = ['cat', 'dog', 'mouse']
for i in range(len(spam)):
print(spam[i])
You may only need for i in spam: instead of the above code. But this applies to a situation where you need an index in the body of the loop, and not just the value itself.
An attempt was made to change a string value. (Error message: "TypeError: 'str' object does not support item assignment" )
Strings are an immutable data type.
Example code with error:
spam = 'I have a pet
cat
.'
spam[13] = 'r'
print(spam)
Correct option :
spam = 'I have a pet cat.'
spam = spam[:13] + 'r' + spam[14:]
print(spam)
An attempt was made to concatenate a non-string value into a string value. (Error message: "TypeError: Can't convert 'int' object to str implicitly" )
Example code with error:
numEggs
= 12
print('I have ' + numEggs + ' eggs.')
Correct option:
numEggs = 12
print('I have ' + str(numEggs) + ' eggs.')
... More:
numEggs = 12
print('I have %s eggs.' % (numEggs))
Omitting quotation marks at the beginning or end of a string value. (Error message: "SyntaxError: EOL while scanning string literal" )
Example code with error:
print(Hello!')
… more:
print('Hello!)
...another example:
myName = 'Al'
print('My name is ' + myName + . How are you?')
There is a typo in a variable or function name. (Error message: "NameError: name 'fooba' is not defined" )
Example code with error:
foobar = 'Al'
print('My name is ' + fooba)
… more:
spam = ruond(4.2)
...another example:
spam = Round(4.2)
There is a typo in the method name. (Error message: "AttributeError: 'str' object has no attribute 'lowerr'" )
Example code with error:
spam = 'THIS IS IN LOWERCASE.'
spam = spam.lowerr()
Out of bounds of the array. (Error message: "IndexError: list index out of range" )
Example code with error:
spam = ['cat', 'dog', 'mouse']
print(spam[6])
There are only three elements in the given array, and the element with index 6 is called for printing.
Using a non-existent dictionary key. (Error message: "KeyError: 'spam'" )
Example code with error:
spam = {'cat': 'Zophie', 'dog': 'Basil', 'mouse': 'Whiskers'}
print('The name of my pet zebra is ' + spam['zebra'])
Trying to use Python keywords as a variable. (Error message: "SyntaxError: invalid syntax" )
Python keywords (also called reserved words) cannot be used to name variables. The error will be with the following code:
class = 'algebra'
Ключевые слова Python 3: and, as, assert, break, class, continue, def, del, elif, else, except, False, finally, for, from, global , if, import, in, is, lambda, None, nonlocal, not, or, pass, raise, return, True, try, while, with, yield .
Using the extended assignment operator for a new variable. (Error message: "NameError: name 'foobar' is not defined" )
Don't assume that variables start with a value such as 0 or the empty string. An expression with an extended operator like spam += 1 is equivalent to spam = spam + 1. This means that there must be some value in spam to begin with.
Example code with error:
spam = 0
spam += 42
eggs += 42
Using local variables (with the same name as a global variable ) in a function before assigning a local variable. (Error message: "UnboundLocalError: local variable 'foobar' referenced before assignment" )
Using a local variable in a function whose name is the same as a global variable is quite difficult. The rule is that if a variable in a function is ever assigned anything, it is always a local variable when used within that function. Otherwise it is a global variable inside this function.
This means that you cannot use it as a global variable in a function until it has been assigned.
Example code with error:
someVar = 42
def myFunction():
print(someVar)
someVar = 100
myFunction()
Trying to use range() to create a list of integers. (Error message: "TypeError: 'range' object does not support item assignment" )
Sometimes you need a list of integer values in order, so range() seems like a good way to create that list. However, you must remember that range() returns a "range object" and not the actual value of the list.
Example code with error:
spam = range(10)
spam[4] = -1
Correct option:
spam = list(range(10))
spam[4] = -1
(This works in Python 2 because Python 2's range() returns a list of values. But if you try it in Python 3, you'll see an error.)
There is no ++ increment or -- decrement operator. (Error message: "SyntaxError: invalid syntax" )
If you are used to other programming languages such as C++, Java or PHP, you try to increment or decrement a variable using ++ or --. There are no such operators in Python.
Example code with error:
spam = 0
spam++
Correct option:
spam = 0
spam += 1
They also often forget to add self as the first parameter to the method. (Error message: " TypeError : TypeError: myMethod() takes no arguments (1 given) ")
Example code with error:
class
Foo
():
def myMethod():
print('Hello!')
a = Foo()
a.myMethod()
The correct way would be:
class Foo():
def myMethod(self):
print('Hello!')
a = Foo()
a.myMethod()
Related Articles:
Installing and configuring Odoo 16 and Pycharm on Ubuntu 22.04
ODOO: remove from database a deleted module